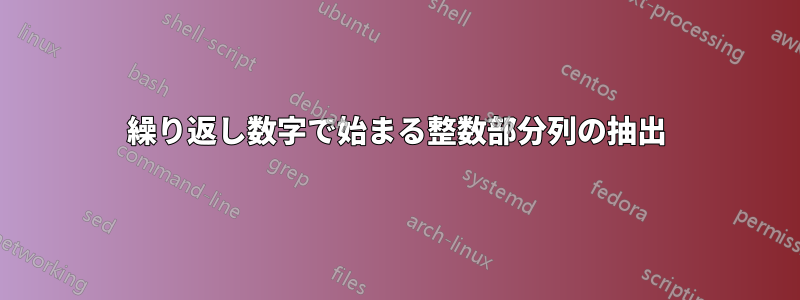
整数の列が 1 つだけ含まれているファイルがあります。このファイルから、同じ数字が 2 回続けて始まり、長さが 12 個の整数 (重複する部分列を含む) であるすべての連続した部分列 (つまり、連続した順序で発生する部分列) のリストを抽出したいと考えています。
さらに、ファイル内の整数以外の行は無視/削除する必要があり、12 個の整数に達する前にシーケンスが入力の末尾に達した場合は、短縮されたシーケンスが出力される必要があります。
たとえば、入力ファイルに次のデータが含まれているとします。
1
junk
1
1
2
3
4
4
5
6
7
8
9
10
11
12
13
14
15
15
16
次に、ソリューションによって次の出力が生成されるはずです。
1 1 1 2 3 4 4 5 6 7 8 9
1 1 2 3 4 4 5 6 7 8 9 10
4 4 5 6 7 8 9 10 11 12 13 14
15 15 16
junk
行と空行は無視され、最初の 3 行は連続したものとして扱われることに注意してください1
。
答え1
以下は、必要なことを実行する Python スクリプトです。
#!/usr/bin/env python2
# -*- coding: ascii -*-
"""extract_subsequences.py"""
import sys
import re
# Open the file
with open(sys.argv[1]) as file_handle:
# Read the data from the file
# Remove white-space and ignore non-integers
numbers = [
line.strip()
for line in file_handle.readlines()
if re.match("^\d+$", line)
]
# Set a lower bound so that we can output multiple lists
lower_bound = 0
while lower_bound < len(numbers)-1:
# Find the "start index" where the same number
# occurs twice at consecutive locations
start_index = -1
for i in range(lower_bound, len(numbers)-1):
if numbers[i] == numbers[i+1]:
start_index = i
break
# If a "start index" is found, print out the two rows
# values and the next 10 rows as well
if start_index >= lower_bound:
upper_bound = min(start_index+12, len(numbers))
print(' '.join(numbers[start_index:upper_bound]))
# Update the lower bound
lower_bound = start_index + 1
# If no "start index" is found then we're done
else:
break
データが というファイルにあるとしますdata.txt
。その場合、このスクリプトを次のように実行できます。
python extract_subsequences.py data.txt
入力ファイルがdata.txt
次のようになっているとします。
1
1
1
2
3
4
5
6
7
8
9
10
11
12
出力は次のようになります。
1 1 1 2 3 4 5 6 7 8 9 10
1 1 2 3 4 5 6 7 8 9 10 11
出力をファイルに保存するには、出力リダイレクトを使用します。
python extract_subsequences.py data.txt > output.txt
答え2
AWK
アプローチ:
最初に遭遇した 2 つの同一の連続した数字のみを考慮します。つまり、複数の抽出に適していますが、処理されたスライスの下の 10 桁のシーケンス内に 2 つの同一の連続した数字が含まれる可能性があるという条件は考慮されません。
awk 'NR==n && $1==v{ print v ORS $1 > "file"++c; tail=n+11; next }
{ v=$1; n=NR+1 }NR<tail{ print > "file"c }' file
答え3
最初のバリエーション - O(n)
awk '
/^[0-9]+$/{
arr[cnt++] = $0;
}
END {
for(i = 1; i < cnt; i++) {
if(arr[i] != arr[i - 1])
continue;
last_element = i + 11;
for(j = i - 1; j < cnt && j < last_element; j++) {
printf arr[j] " ";
}
print "";
}
}' input.txt
2番目のバリエーション - O(n * n)
awk '
BEGIN {
cnt = 0;
}
/^[0-9]+$/{
if(prev == $0) {
arr[cnt] = prev;
cnt_arr[cnt]++;
cnt++;
}
for(i = 0; i < cnt; i++) {
if(cnt_arr[i] < 12) {
arr[i] = arr[i] " " $0;
cnt_arr[i]++;
}
}
prev = $0;
}
END {
for(i = 0; i < cnt; i++)
print arr[i];
}' input.txt
出力
1 1 1 2 3 4 4 5 6 7 8 9
1 1 2 3 4 4 5 6 7 8 9 10
4 4 5 6 7 8 9 10 11 12 13 14
15 15 16