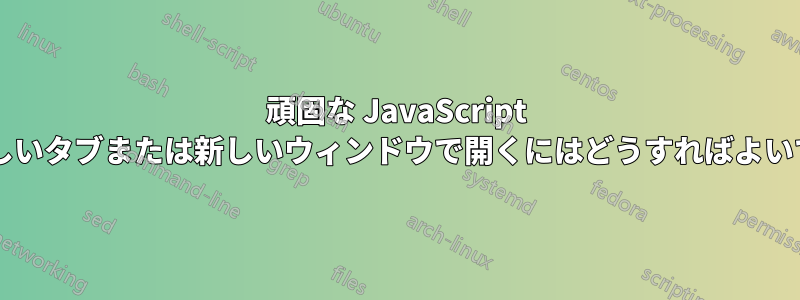
一部の Web サイトでは、「クリエイティブな」(JavaScript の?) ハイパーリンクが使用されているため、Ctrl キーを押しながらクリックしたり、中クリックしてリンクを新しいタブで開くなどのブラウザの機能が損なわれます。
よくある例として、taleo HRウェブサイト http://www.rogers.com/web/Careers.portal?_nfpb=true&_pageLabel=C_CP&_page=9
何を試しても、リンクを普通にクリックするだけで、新しいウィンドウで開くことができません。これを回避する方法はありますか?
答え1
あなたの質問は Taleo に特有のものなので、私の答えも同様になります :)
必要なことを実行する UserScript をコーディングしました。このスクリプトは、すべての JavaScript リンクを通常のリンクに置き換えます。そのため、必要に応じてリンクをクリックしたり、新しいタブで開いたりすることができます。
// ==UserScript==
// @name Taleo Fix
// @namespace https://github.com/raphaelh/taleo_fix
// @description Taleo Fix Links
// @include http://*.taleo.net/*
// @include https://*.taleo.net/*
// @version 1
// @grant none
// ==/UserScript==
function replaceLinks() {
var rows = document.getElementsByClassName("titlelink");
var url = window.location.href.substring(0, window.location.href.lastIndexOf("/") + 1) + "jobdetail.ftl";
for (var i = 0; i < rows.length; i++) {
rows[i].childNodes[0].href = url + "?job=" + rows[i].parentNode.parentNode.parentNode.parentNode.parentNode.id;
}
}
if (typeof unsafeWindow.ftlPager_processResponse === 'function') {
var _ftlPager_processResponse = unsafeWindow.ftlPager_processResponse;
unsafeWindow.ftlPager_processResponse = function(f, b) {
_ftlPager_processResponse(f, b);
replaceLinks();
};
}
if (typeof unsafeWindow.requisition_restoreDatesValues === 'function') {
var _requisition_restoreDatesValues = unsafeWindow.requisition_restoreDatesValues;
unsafeWindow.requisition_restoreDatesValues = function(d, b) {
_requisition_restoreDatesValues(d, b);
replaceLinks();
};
}
こちらからご覧いただけます:https://github.com/raphaelh/taleo_fix/blob/master/Taleo_Fix.user.js
答え2
はい。独自のスクリプトを書くことができます。グリースモンキー(Firefox) またはタンパーモンキー(クロム)
あなたが言及した例では、この Tampermonkey UserScript は、検索結果内のすべての JavaScript リンクを新しいタブ/ウィンドウで開くように設定します (これはブラウザの設定によって異なりますが、私の場合はタブです)。
// ==UserScript==
// @name open links in tabs
// @match http://rogers.taleo.net/careersection/technology/jobsearch.ftl*
// ==/UserScript==
document.getElementById('ftlform').target="_blank"
より汎用的なバージョンを記述することもできますが、他のユーザビリティを損なうことなくすべての JavaScript リンクに対してこの機能を有効にすることは困難です。
中間的な方法としては、 のイベント ハンドラーを設定しCtrl、キーが押されている限り、すべてのフォームのターゲットを一時的に "_blank" に設定することが考えられます。
答え3
onclick="document.location='some_url'"
これは、属性を持つ任意の要素を要素内にラップし<a href=some_url>
、 を削除する別のユーザー スクリプトですonclick
。
これは特定のサイト向けに書いたものですが、一般的な内容なので他の人にも役立つかもしれません。@マッチURLは下記。
これは、リンクが AJAX 呼び出しによって読み込まれたときに機能するため、MutationObserver が使用されます。
// ==UserScript==
// @name JavaScript link fixer
// @version 0.1
// @description Change JavaScript links to open in new tab/window
// @author EM0
// @match http://WHATEVER-WEBSITE-YOU-WANT/*
// @grant none
// ==/UserScript==
var modifyLink = function(linkNode) {
// Re-create the regex every time, otherwise its lastIndex needs to be reset
var linkRegex = /document\.location\s*=\s*\'([^']+)\'/g;
var onclickText = linkNode.getAttribute('onclick');
if (!onclickText)
return;
var match = linkRegex.exec(onclickText);
if (!match) {
console.log('Failed to find URL in onclick text ' + onclickText);
return;
}
var targetUrl = match[1];
console.log('Modifying link with target URL ' + targetUrl);
// Clear onclick, so it doesn't match the selector, before modifying the DOM
linkNode.removeAttribute('onclick');
// Wrap the original element in a new <a href='target_url' /> element
var newLink = document.createElement('a');
newLink.href = targetUrl;
var parent = linkNode.parentNode;
newLink.appendChild(linkNode);
parent.appendChild(newLink);
};
var modifyLinks = function() {
var onclickNodes = document.querySelectorAll('*[onclick]');
[].forEach.call(onclickNodes, modifyLink);
};
var observeDOM = (function(){
var MutationObserver = window.MutationObserver || window.WebKitMutationObserver;
return function(obj, callback) {
if (MutationObserver) {
var obs = new MutationObserver(function(mutations, observer) {
if (mutations[0].addedNodes.length || mutations[0].removedNodes.length)
callback();
});
obs.observe(obj, { childList:true, subtree:true });
}
};
})();
(function() {
'use strict';
observeDOM(document.body, modifyLinks);
})();