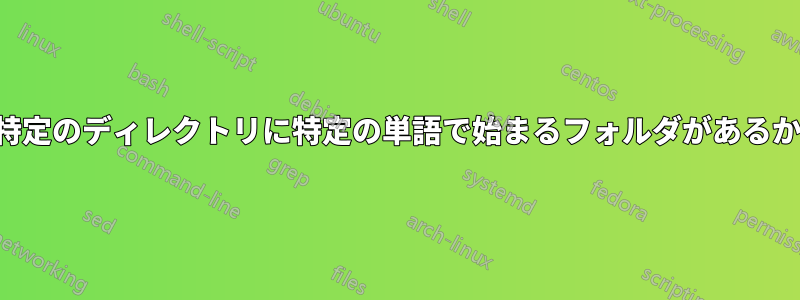
特定のディレクトリ内で特定の単語で始まるサブディレクトリをチェックするスクリプトを作成しています。
これまでの私のスクリプトは次のとおりです。
#!/bin/bash
function checkDirectory() {
themeDirectory="/usr/share/themes"
iconDirectory="/usr/share/icons"
# I don't know what to put for the regex.
regex=
if [ -d "$themeDirectory/$regex" && -d "$iconDirectory/$regex" ]; then
echo "Directories exist."
else
echo "Directories don't exist."
fi
}
regex
では、特定のディレクトリに特定の単語で始まるフォルダーがあるかどうかを確認するには、どのように使用すればよいでしょうか?
答え1
-d
正規表現は受け付けませんが、ファイル名は受け付けます。単純なプレフィックスだけをチェックしたい場合は、ワイルドカードで十分です。
exists=0
shopt -s nullglob
for file in "$themeDirectory"/word* "$iconDirectory"/* ; do
if [[ -d $file ]] ; then
exists=1
break
fi
done
if ((exists)) ; then
echo Directory exists.
else
echo "Directories don't exist."
fi
nullglob
一致するものがない場合、ワイルドカードは空のリストに展開されます。 大きなスクリプトでは、サブシェルで値を変更するか、必要がなくなったら古い値を元に戻します。
答え2
特定のパターン/プレフィックスに一致するディレクトリのみを検索したい場合は、次のようにすればよいと思いますfind
:
find /target/directory -type d -name "prefix*"
または、すぐにサブディレクトリ:
find /target/directory -maxdepth 1 -type d -name "prefix*"
もちろん、-regex
実際の正規表現の一致が必要な場合もあります。(注意: -maxdepth が gnu の仕様かどうかは覚えていません。)
(更新) そうです、if ステートメントが必要でした。Find は常にゼロを返すので、戻り値を使用して何かが見つかったかどうかを確認することはできません (grep とは異なります)。ただし、たとえば行数をカウントすることはできます。出力をパイプしてwc
カウントを取得し、ゼロでないかどうかを確認します。
if [ $(find /target/directory -type d -name "prefix*" | wc -l ) != "0" ] ; then
echo something was found
else
echo nope, didn't find anything
fi
答え3
変数の名前はregex
適切に選択されていませんが、値を のように設定することを検討してください"$1"
。次の手順では、ステートメントを次のようにregex="$1"
変更します。if
if [ -d "$themeDirectory/$regex" && -d "$iconDirectory/$regex" ]; then
に
if [ -d "$themeDirectory/$regex" ] && [ -d "$iconDirectory/$regex" ]; then
スクリプトは次のようになります。
function checkDirectory() {
themeDirectory="/usr/share/themes"
iconDirectory="/usr/share/icons"
# I don't know what to put for the regex.
regex="$1"
if [ -d "$themeDirectory/$regex" ] && [ -d "$iconDirectory/$regex" ]; then
echo "Directories exist."
else
echo "Directories don't exist."
fi
}
シェルからスクリプトをソースするには、次の操作を実行します。
. /path/to/script
これで関数は使用可能になりました:
checkDirectory test
Directories don't exist.