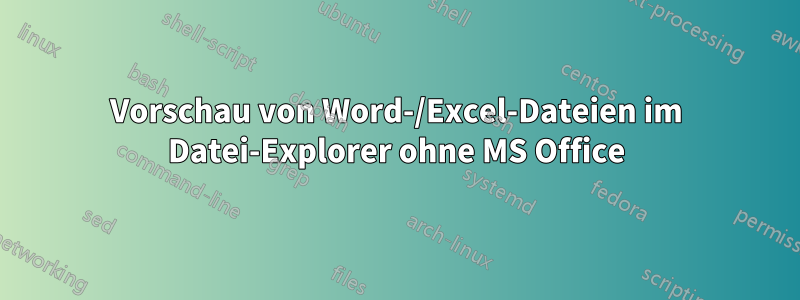
Ich bin Dozent an einer Universität. Wir sind gezwungen, WPS Office zu verwenden, da das Institut die Kosten für MS Office nicht tragen kann. Daher haben wir die Standardfunktion in Windows verloren, um Word-/Excel-Dateien in der Vorschau anzuzeigen, nachdem wir den Vorschaubereich im Windows-Datei-Explorer aktiviert haben. Die letzten 2 Tage habe ich damit verbracht, nach einem alternativen Datei-Explorer zu suchen, aber obwohl einige Anbieter die Vorschau versprechen, ist sie entweder für Office-Dokumente nicht verfügbar oder verfügt überhaupt nicht über die Vorschaufunktion.
Die Fakultät benötigt die Vorschau dringend, da wir oft viele Word-Dateien durchgehen, indem wir sie einfach im Windows-Datei-Explorer anklicken oder mit den Pfeiltasten durch sie navigieren.
Bitte schlagen Sie eine funktionierende Lösung vor
Antwort1
Da ich WinForms-Anwendungen entwickeln kann, habe ich verschiedene Lösungen ausprobiert.
Die meisten davon waren nicht kostenlos und daher nicht verwendbar. Dann hat mir der Pandoc-Konverter geholfen, aber er war immer noch nicht sehr gut.
Also wechselte ich zur Community Edition von Syncfusion. Sie ist bereits kostenlos und ihre Bibliotheken ermöglichen die Konvertierung aller gängigen Textverarbeitungsformate. Das Ergebnis war dasselbe wie bei anderen nicht kostenlosen Lösungen. Ich teile die Quelle hier, falls jemand sie braucht (es handelt sich um eine Winforms-Code-Behind-Datei). Sie ermöglicht die Vorschau aller gängigen Textverarbeitungsformate (.doc, .docx, .rtf, .dot, .odt). Excel- oder PPT-Formate werden nicht unterstützt, aber jeder kann sie erweitern, um auch diese einzuschließen.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Diagnostics;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading;
using System.Windows.Forms;
using CefSharp;
using CefSharp.WinForms;
using Microsoft.VisualBasic.FileIO;
using Syncfusion.DocIO;
using Syncfusion.DocIO.DLS;
using Syncfusion.DocToPDFConverter;
using Syncfusion.OfficeChart;
using Syncfusion.OfficeChartToImageConverter;
using Syncfusion.Pdf;
using NPOI;
using NPOI.HSSF.UserModel;
using NPOI.SS.UserModel;
using NPOI.XSSF.UserModel;
namespace DocPreViewerNew
{
public partial class Form1 : Form
{
public ChromiumWebBrowser wb;
List<string> AllFiles = new List<string>();
List<string> AllNamesOnly = new List<string>();
string SelectedFile;
string NameOnly;
int ResizeUnit = 40;
string[] SourcePath;
int PDFZoomUnit = 100;
string XLPath = "";
public Form1()
{
InitializeComponent();
InitializeChromium();
}
private void Form1_Load(object sender, EventArgs e)
{
this.KeyPreview = true;
//lbFiles.Width = Convert.ToInt32(this.Width * 0.23);
//panel2.Location = new Point(lbFiles.Location.X +Convert.ToInt32(this.Width * 0.23)+5, panel2.Location.Y);
//panel2.Width = Convert.ToInt32(this.Width*0.75);
if (Properties.Settings.Default.PreviewMode.Equals("HTML")) rbHTML.Checked = true;
else rbPDF.Checked = true;
PDFZoomUnit = Convert.ToInt32(txtPDFZoom.Text.Trim());
}
private void lbFiles_SelectedIndexChanged(object sender, EventArgs e)
{
try
{
PDFZoomUnit = Convert.ToInt32(txtPDFZoom.Text.Trim());
wb.LoadUrl("about:blank"); lblFCount.Text = "";
if (lbFiles.SelectedIndices.Count == 1)
{
// Two string lists
SelectedFile = AllFiles[lbFiles.SelectedIndex];
NameOnly = AllNamesOnly[lbFiles.SelectedIndex];
lblFCount.Text = "File No: " + (lbFiles.SelectedIndex+1).ToString();
if (NameOnly.EndsWith(".docx") || NameOnly.EndsWith(".doc") || NameOnly.EndsWith(".rtf"))
{
//Loads the template document
WordDocument document = new WordDocument(SelectedFile, FormatType.Automatic);
if (rbHTML.Checked == true)
{
//Saves the document as Html file
document.Save(Environment.CurrentDirectory + "\\" + "output.html", FormatType.Html);
wb.LoadUrl(Environment.CurrentDirectory + "\\" + "output.html");
}
else
{
//Initializes the ChartToImageConverter for converting charts during Word to pdf conversion
document.ChartToImageConverter = new ChartToImageConverter();
//Sets the scaling mode for charts (Normal mode reduces the Pdf file size)
document.ChartToImageConverter.ScalingMode = ScalingMode.Normal;
//Creates an instance of the DocToPDFConverter - responsible for Word to PDF conversion
DocToPDFConverter converter = new DocToPDFConverter();
//Sets true to enable the fast rendering using direct PDF conversion.
converter.Settings.EnableFastRendering = true;
//Converts Word document into PDF document
PdfDocument pdfDocument = converter.ConvertToPDF(document);
//Saves the PDF file to file system
pdfDocument.Save(Environment.CurrentDirectory + "\\" + "output.pdf");
//Closes the instance of document objects
wb.LoadUrl(Environment.CurrentDirectory + "\\" + "output.pdf#zoom=" + PDFZoomUnit.ToString() + "&toolbar=0");
pdfDocument.Close(true);
//Closes the document
document.Close();
}
}
else if (NameOnly.EndsWith(".pdf"))
{
wb.LoadUrl(SelectedFile + "#zoom=" + PDFZoomUnit.ToString() + "&toolbar=0");
}
else if (NameOnly.EndsWith(".txt"))
wb.LoadUrl(SelectedFile);
else
{
File.WriteAllText(Environment.CurrentDirectory + "\\" + "error1.html", "<h3>** Some Error **</h3><h4>Format not supported</h4>");
wb.LoadUrl(Environment.CurrentDirectory + "\\" + "error1.html");
}
}
else if (lbFiles.SelectedIndices.Count > 1)
{
lblFCount.Text = "Total Selected: " + lbFiles.SelectedIndices.Count.ToString();
}
}
catch(Exception ex)
{
File.WriteAllText(Environment.CurrentDirectory + "\\" + "error1.html", "<h3>** Some Error **</h3><h4>" + ex.Message + "</h4>");
wb.LoadUrl(Environment.CurrentDirectory + "\\" + "error1.html");
}
}
private void btnMoveSelected_Click(object sender, EventArgs e)
{
}
//********
#region UtilityFuntions
private void lbFiles_DoubleClick(object sender, EventArgs e)
{
StartMaximized(AllFiles[lbFiles.SelectedIndex]);
}
private void lbFiles_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.Enter)
StartMaximized(AllFiles[lbFiles.SelectedIndex]);
else if (e.KeyCode == Keys.Delete)
{
try
{
if (lbFiles.SelectedIndex != -1)
{
var confirmResult = MessageBox.Show("Are you sure to delete this item ??", "Confirm Delete!!", MessageBoxButtons.YesNo);
if (confirmResult == DialogResult.Yes)
{
wb.LoadUrl("about:blank");
FileSystem.DeleteFile(AllFiles[lbFiles.SelectedIndex], UIOption.OnlyErrorDialogs, RecycleOption.SendToRecycleBin);
AllFiles.RemoveAt(lbFiles.SelectedIndex);
AllNamesOnly.RemoveAt(lbFiles.SelectedIndex);
lbFiles.SelectedIndexChanged -= lbFiles_SelectedIndexChanged;
lbFiles.Items.RemoveAt(lbFiles.SelectedIndex);
lbFiles.SelectedIndexChanged += lbFiles_SelectedIndexChanged;
}
}
}
catch (OperationCanceledException) { }
}
}
private void Form1_DragDrop(object sender, DragEventArgs e)
{
try
{
RefreshEveryThing();
btnXL.Enabled = true; btnMarks.Enabled = false;
SourcePath = (string[])e.Data.GetData(DataFormats.FileDrop, false);
if (SourcePath.Length == 1)
{
XLPath = "";
AllFiles = Directory.GetFiles(SourcePath[0]).ToList();
string tmp = "";
foreach (string f in AllFiles)
{
tmp = f.Substring(f.LastIndexOf(@"\") + 1);
AllNamesOnly.Add(tmp);
lbFiles.Items.Add(tmp);
}
lbFiles.Sorted = true;
XLPath = SourcePath[0];
}
}
catch { }
}
private void Form1_DragEnter(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(DataFormats.FileDrop))
e.Effect = DragDropEffects.Copy;
else
e.Effect = DragDropEffects.None;
}
private void StartMaximized(string FullFileName)
{
Process P = new Process();
P.StartInfo.UseShellExecute = true;
P.StartInfo.WindowStyle = ProcessWindowStyle.Maximized;
P.StartInfo.FileName = FullFileName;
P.Start();
}
private void Sleeep(int s)
{
Thread.Sleep(TimeSpan.FromSeconds(s));
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
Cef.Shutdown();
if (rbPDF.Checked == true) Properties.Settings.Default.PreviewMode = "PDF";
else Properties.Settings.Default.PreviewMode = "HTML";
Properties.Settings.Default.Save();
}
private void btnDec_Click(object sender, EventArgs e)
{
Point p = panel2.Location;
lbFiles.Width -= ResizeUnit;
panel2.Location = new Point(p.X - ResizeUnit, p.Y);
//lblFCount.Location = new Point(p.X - ResizeUnit, lbFiles.Location.Y);
panel2.Width += ResizeUnit;
}
private void btnInc_Click(object sender, EventArgs e)
{
Point p = panel2.Location;
lbFiles.Width += ResizeUnit;
panel2.Location = new Point(p.X + ResizeUnit, p.Y);
//lblFCount.Location = new Point(p.X + ResizeUnit, lbFiles.Location.Y);
panel2.Width -= ResizeUnit;
}
private void RefreshEveryThing()
{
try
{
AllFiles.Clear();
AllNamesOnly.Clear();
lbFiles.Items.Clear();
SourcePath = null;
SelectedFile = "";
NameOnly = "";
wb.LoadUrl("about:blank");
if (File.Exists(Environment.CurrentDirectory + "\\" + "output.html"))
FileSystem.DeleteFile(Environment.CurrentDirectory + "\\" + "output.html", UIOption.OnlyErrorDialogs, RecycleOption.DeletePermanently);
if (File.Exists(Environment.CurrentDirectory + "\\" + "output.pdf"))
FileSystem.DeleteFile(Environment.CurrentDirectory + "\\" + "output.pdf", UIOption.OnlyErrorDialogs, RecycleOption.DeletePermanently);
//if (SourcePath!=null && SourcePath.Length == 1)
//{
// AllFiles = Directory.GetFiles(SourcePath[0]).ToList();
// string tmp = "";
// foreach (string f in AllFiles)
// {
// tmp = f.Substring(f.LastIndexOf(@"\") + 1);
// AllNamesOnly.Add(tmp);
// lbFiles.Items.Add(tmp);
// }
//}
}
catch { }
}
public void InitializeChromium()
{
CefSettings settings = new CefSettings();
// Initialize cef with the provided settings
Cef.Initialize(settings);
// Create a browser component
wb = new ChromiumWebBrowser("");
// Add it to the form and fill it to the form window.
this.panel2.Controls.Add(wb);
wb.Dock = DockStyle.Fill;
}
#endregion
private void btnMarks_Click(object sender, EventArgs e)
{
if (lbFiles.SelectedIndex != -1)
{
Marks frmMarks = new Marks();
frmMarks.ID = lbFiles.SelectedItem.ToString();
frmMarks.XLFILE = XLPath;
frmMarks.ShowDialog();
if (frmMarks.MARKSENTERED == true)
lblFCount.Text = "Marks entered successfully";
else
lblFCount.Text = "**Problem entering Marks**";
frmMarks.Close();
}
}
private void btnXL_Click(object sender, EventArgs e)
{
HSSFWorkbook hssfwb=null;
try
{
if (Directory.Exists(SourcePath[0]))
{
OpenFileDialog XL = new OpenFileDialog();
if (XLPath != String.Empty) XL.InitialDirectory = XLPath;
XL.Title = "Select Marks Sheet File";
XL.Filter = "Excel Files|*.xls;*.xlsx";
if (XL.ShowDialog() == DialogResult.OK) XLPath = XL.FileName;
//Check old Excel File
if (XLPath.EndsWith(".xls"))
{
using (FileStream file = new FileStream(XLPath, FileMode.Open, FileAccess.Read))
{
hssfwb = new HSSFWorkbook(file);
file.Close();
}
ISheet sheet = hssfwb.GetSheetAt(0);
try
{
if (sheet.GetRow(0).GetCell(0).StringCellValue != "StudentId" || sheet.GetRow(0).GetCell(1).StringCellValue != "Marks" || sheet.GetRow(0).GetCell(2).StringCellValue != "Comments")
{
MessageBox.Show("First sheet must have these three columns:\n\nStudentId Marks Comments");
}
else btnMarks.Enabled = true;
}
catch (NullReferenceException) { MessageBox.Show("First sheet must have these three columns:\n\nStudentId Marks Comments"); }
}
}
}
catch { }
finally
{
if (hssfwb!=null) hssfwb.Close();
btnXL.Enabled = !btnMarks.Enabled;
}
}
private void Form1_KeyDown(object sender, KeyEventArgs e)
{
if (e.Alt && e.KeyCode == Keys.M)
{
btnMarks.PerformClick();
//btncash_Click(null, null);
}
}
} // Name space and class ends
}