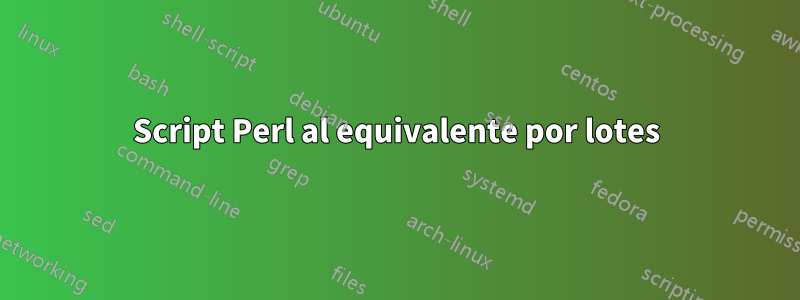
Creé un archivo PERL, pero como es bastante simplista, parece un poco "exagerado" enviar a las personas 16 MB de PERL en una memoria USB solo para ejecutar un programa, por lo que me pregunto si un archivo por lotes de DOS más pequeño podría funcionar. La parte importante -EXIFTOOL- se puede ejecutar desde CMD (incluiré el código más adelante).
Aquí está el archivo txt de la hora:
ABC|10:15
DEF|10:30
XYZ|10:40
El script carga las fotos, las pone en orden numérico (en lugar de 1, 10, 2, 20 que da la clasificación básica) y, usando EXIF, encuentra cuándo se tomó la foto. Quita los dos valores superiores del archivo. "Si hora> A && hora <B, mover a la carpeta" Entonces, una marca de tiempo de 10:16, 10:20, 10:25 se mueve a ABC.
Sin embargo, la marca de tiempo de las 10:33 está por encima de B... por lo que convierte a B en la nueva A y obtiene la próxima hora del archivo de texto (10:40). Así que ahora mueve cualquier marca de tiempo entre las 10:30 y las 10:40 a la carpeta DEF. (Agrego una entrada ficticia al final del archivo de tiempo para que X+1 no intente leer más allá del final del archivo. El bucle está configurado en @data-1. Entonces, si el archivo tiene 12 líneas de largo, recorre a 11, y lee x+1 de la línea ficticia al final... que es AHORA, y ninguna foto tendrá esa marca de tiempo)
¿Cree que estos bucles/directorios de lectura/si las declaraciones podrían condensarse en un simple archivo por lotes?
#!/usr/bin/perl
use v5.10;
use strict;
use warnings;
my $photoFolder='../../EventsSoft/photos';
use Image::ExifTool;
use File::Copy;
use Time::Local;
### IMPORTANT ###
my $mainFolder='AHS_school';
### IMPORTANT ###
if (!defined $mainFolder || $mainFolder eq ''){
mkdir $photoFolder.'/temp';
$mainFolder='temp';
}
elsif (not -e $photoFolder.'/'.$mainFolder){
mkdir $photoFolder.'/'.$mainFolder;
}
my $exif=new Image::ExifTool;
my ($sec,$min,$hour,$mday,$mon,$year,$wday,$yday,$isdst,$currentFile,$nextFile,$barcode,$dummy);
my @timePic;
my @checkPics;
opendir my $ht, $photoFolder or die "Could not open photo folder for reading '$!'\n";
my @ht = grep {/\.jpg$/} readdir $ht; # Only keep the JPG files
closedir $ht;
@checkPics = map { $_->[0] } # Sorts the files so run 1,2,3 not 1,11,12
sort { $a->[1] <=> $b->[1] }
map { [$_, $_=~/(\d+)/] } @ht;
for (my $x=0; $x<@checkPics; $x++){
$exif->ExtractInfo($photoFolder.'/'.'photo'.$x.'.jpg');
$timePic[$x]=$exif->GetValue('CreateDate');
}
open (my $fh, '<', $photoFolder.'/photog1.dat') or die "Could not open photog1.dat for reading '$!'\n";
my @data=<$fh>;
close($fh);
chomp @data;
($sec,$min,$hour,$mday,$mon,$year,$wday,$yday,$isdst) = localtime(time);
if ($mon eq 11){
$mon=0;
}
else{
$mon++;
}
my $str = sprintf ("%02d:%02d %02d:%02d:%02d", $mon, $mday, $hour, $min, $sec);
my $fakeTime='XXXXXX|'.($year+1900).':'.$str;
push (@data,$fakeTime); # Saves time in ISO format
# @data holds the time barcode scanned in the format XXXAGK|2019-03-15 11:14:00
# @checkPics holds the image filename
# @timePic holds time photo was taken
# The fake entry stops reading beyond end of file with ($y+1) option below. Np photos would have been taken at this time/date
for (my $x=0; $x<@data-1; $x++){
($barcode,$currentFile)=split('\|',$data[$x]); # Gets the top value off data, and gets the barcode
($dummy,$nextFile)=split('\|',$data[($x+1)]); # Gets the next value down - files to be below this value
for (my $y=0;$y<@timePic; $y++){
if ($timePic[$y] eq 0){
next;
}
if ($timePic[$y] lt $nextFile){
mkdir $photoFolder.'/'.$mainFolder.'/'.$barcode;
copy ($photoFolder.'/'.$checkPics[$y], $photoFolder.'/'.$mainFolder.'/'.$barcode.'/'.$checkPics[$y]) or die "Copy failed line 70 '$!'\n";
$timePic[$y]=0;
}
if ($timePic[$y] gt $nextFile){
last;
}
}
}
## Because the above loop is @data-1; we do not get to the last entry, so need one final loop
my $picEnd=@data;
($barcode,$dummy)=split('\|',$data[$picEnd-2]); # Gets the final barcode
for (my $y=0;$y<@timePic; $y++){
if ($timePic[$y] eq 0){
next;
}
mkdir $photoFolder.'/'.$mainFolder.'/'.$barcode;
copy ($photoFolder.'/'.$checkPics[$y], $photoFolder.'/'.$mainFolder.'/'.$barcode.'/'.$checkPics[$y]) or die "Copy failed line 86'$!'\n";
}
if ($mainFolder eq 'temp'){
rename $photoFolder.'/temp',$photoFolder.'/!! TEMP !!';
}
say "All photos have been moved to their respective folders";