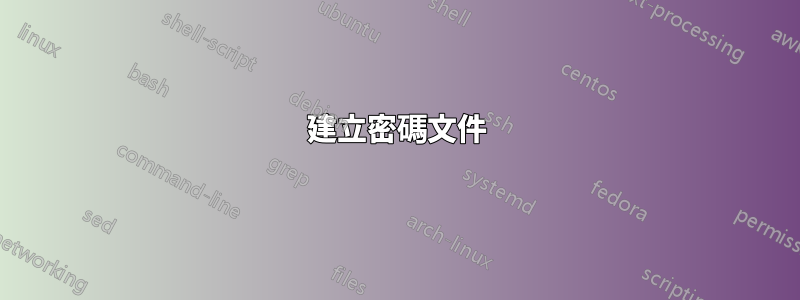
我有一個腳本,用於更改 Microsoft 的本機管理員密碼。 https://support.microsoft.com/en-us/kb/2962486?wa=wsignin1.0是我找到它的地方。對於那些懶得點擊的人來說,這是一個腳本:
function Invoke-PasswordRoll
{
<#
.SYNOPSIS
This script can be used to set the local account passwords on remote machines to random passwords. The
username/password/server combination will be saved in a CSV file.
The account passwords stored in the CSV file can be encrypted using a password of the administrators choosing to
ensure clear-text account passwords aren't written to disk.
The encrypted passwords can be decrypted using another function in this file: ConvertTo-CleartextPassword
Function: Invoke-PasswordRoll
Author: Microsoft
Version: 1.0
.DESCRIPTION
This script can be used to set the local account passwords on remote machines to random passwords. The
username/password/server combination will be saved in a CSV file.
The account passwords stored in the CSV file can be encrypted using a password of the administrators choosing to
ensure clear-text account passwords aren't written to disk.
The encrypted passwords can be decrypted using another function in this file: ConvertTo-CleartextPassword
.PARAMETER ComputerName
An array of computers to run the script against using PowerShell remoting.
.PARAMETER LocalAccounts
An array of local accounts whose password should be changed.
.PARAMETER TsvFileName
The file to output the username/password/server combinations to.
.PARAMETER EncryptionKey
A password to encrypt the TSV file with. Uses AES encryption. Only the passwords stored in the TSV file will be
encrypted, the username and servername will be clear-text.
.PARAMETER PasswordLength
The length of the passwords which will be randomly generated for local accounts.
.PARAMETER NoEncryption
Do not encrypt the account passwords stored in the TSV file. This will result in clear-text passwords being
written to disk.
.EXAMPLE
. .\Invoke-PasswordRoll.ps1 #Loads the functions in this script file
Invoke-PasswordRoll -ComputerName (Get-Content computerlist.txt) -LocalAccounts @
("administrator","CustomLocalAdmin") -TsvFileName "LocalAdminCredentials.tsv" -EncryptionKey "Password1"
Connects to all the computers stored in the file "computerlist.txt". If the local account "administrator" and/or
"CustomLocalAdmin" are present on the system, their password is changed
to a randomly generated password of length 20 (the default). The username/password/server combinations are
stored in LocalAdminCredentials.tsv, and the account passwords are AES encrypted using the password "Password1".
.EXAMPLE
. .\Invoke-PasswordRoll.ps1 #Loads the functions in this script file
Invoke-PasswordRoll -ComputerName (Get-Content computerlist.txt) -LocalAccounts @("administrator") -TsvFileName
"LocalAdminCredentials.tsv" -NoEncryption -PasswordLength 40
Connects to all the computers stored in the file "computerlist.txt". If the local account "administrator" is
present on the system, its password is changed to a random generated
password of length 40. The username/password/server combinations are stored in LocalAdminCredentials.tsv
unencrypted.
.NOTES
Requirements:
-PowerShellv2 or above must be installed
-PowerShell remoting must be enabled on all systems the script will be run against
Script behavior:
-If a local account is present on the system, but not specified in the LocalAccounts parameter, the script will
write a warning to the screen to alert you to the presence of this local account. The script will continue
running when this happens.
-If a local account is specified in the LocalAccounts parameter, but the account does not exist on the computer,
nothing will happen (an account will NOT be created).
-The function ConvertTo-CleartextPassword, contained in this file, can be used to decrypt passwords that are
stored encrypted in the TSV file.
-If a server specified in ComputerName cannot be connected to, PowerShell will output an error message.
-Microsoft advises companies to regularly roll all local and domain account passwords.
#>
[CmdletBinding(DefaultParameterSetName="Encryption")]
Param(
[Parameter(Mandatory=$true)]
[String[]]
$ComputerName,
[Parameter(Mandatory=$true)]
[String[]]
$LocalAccounts,
[Parameter(Mandatory=$true)]
[String]
$TsvFileName,
[Parameter(ParameterSetName="Encryption", Mandatory=$true)]
[String]
$EncryptionKey,
[Parameter()]
[ValidateRange(20,120)]
[Int]
$PasswordLength = 20,
[Parameter(ParameterSetName="NoEncryption", Mandatory=$true)]
[Switch]
$NoEncryption
)
#Load any needed .net classes
Add-Type -AssemblyName "System.Web" -ErrorAction Stop
#This is the scriptblock that will be executed on every computer specified in ComputerName
$RemoteRollScript = {
Param(
[Parameter(Mandatory=$true, Position=1)]
[String[]]
$Passwords,
[Parameter(Mandatory=$true, Position=2)]
[String[]]
$LocalAccounts,
#This is here so I can record what the server name that the script connected to was, sometimes the
DNS records get messed up, it can be nice to have this.
[Parameter(Mandatory=$true, Position=3)]
[String]
$TargettedServerName
)
$LocalUsers = Get-WmiObject Win32_UserAccount -Filter "LocalAccount=true" | Foreach {$_.Name}
#Check if the computer has any local user accounts whose passwords are not going to be rolled by this
script
foreach ($User in $LocalUsers)
{
if ($LocalAccounts -inotcontains $User)
{
Write-Warning "Server: '$($TargettedServerName)' has a local account '$($User)' whos password is
NOT being changed by this script"
}
}
#For every local account specified that exists on this server, change the password
$PasswordIndex = 0
foreach ($LocalAdmin in $LocalAccounts)
{
$Password = $Passwords[$PasswordIndex]
if ($LocalUsers -icontains $LocalAdmin)
{
try
{
$objUser = [ADSI]"WinNT://localhost/$($LocalAdmin), user"
$objUser.psbase.Invoke("SetPassword", $Password)
$Properties = @{
TargettedServerName = $TargettedServerName
Username = $LocalAdmin
Password = $Password
RealServerName = $env:computername
}
$ReturnData = New-Object PSObject -Property $Properties
Write-Output $ReturnData
}
catch
{
Write-Error "Error changing password for user:$($LocalAdmin) on server:
$($TargettedServerName)"
}
}
$PasswordIndex++
}
}
#Generate the password on the client running this script, not on the remote machine. System.Web.Security
isn't available in the .NET Client profile. Making this call
# on the client running the script ensures only 1 computer needs the full .NET runtime installed (as
opposed to every system having the password rolled).
function Create-RandomPassword
{
Param(
[Parameter(Mandatory=$true)]
[ValidateRange(20,120)]
[Int]
$PasswordLength
)
$Password = [System.Web.Security.Membership]::GeneratePassword($PasswordLength, $PasswordLength / 4)
#This should never fail, but I'm putting a sanity check here anyways
if ($Password.Length -ne $PasswordLength)
{
throw new Exception("Password returned by GeneratePassword is not the same length as required.
Required length: $($PasswordLength). Generated length: $($Password.Length)")
}
return $Password
}
#Main functionality - Generate a password and remote in to machines to change the password of local accounts
specified
if ($PsCmdlet.ParameterSetName -ieq "Encryption")
{
try
{
$Sha256 = new-object System.Security.Cryptography.SHA256CryptoServiceProvider
$SecureStringKey = $Sha256.ComputeHash([System.Text.UnicodeEncoding]::Unicode.GetBytes
($EncryptionKey))
}
catch
{
Write-Error "Error creating TSV encryption key" -ErrorAction Stop
}
}
foreach ($Computer in $ComputerName)
{
#Need to generate 1 password for each account that could be changed
$Passwords = @()
for ($i = 0; $i -lt $LocalAccounts.Length; $i++)
{
$Passwords += Create-RandomPassword -PasswordLength $PasswordLength
}
Write-Output "Connecting to server '$($Computer)' to roll specified local admin passwords"
$Result = Invoke-Command -ScriptBlock $RemoteRollScript -ArgumentList @($Passwords, $LocalAccounts,
$Computer) -ComputerName $Computer
#If encryption is being used, encrypt the password with the user supplied key prior to writing to disk
if ($Result -ne $null)
{
if ($PsCmdlet.ParameterSetName -ieq "NoEncryption")
{
$Result | Select-Object Username,Password,TargettedServerName,RealServerName | Export-Csv -
Append -Path $TsvFileName -NoTypeInformation
}
else
{
#Filters out $null entries returned
$Result = $Result | Select-Object Username,Password,TargettedServerName,RealServerName
foreach ($Record in $Result)
{
$PasswordSecureString = ConvertTo-SecureString -AsPlainText -Force -String
($Record.Password)
$Record | Add-Member -MemberType NoteProperty -Name EncryptedPassword -Value (ConvertFrom-
SecureString -Key $SecureStringKey -SecureString $PasswordSecureString)
$Record.PSObject.Properties.Remove("Password")
$Record | Select-Object Username,EncryptedPassword,TargettedServerName,RealServerName |
Export-Csv -Append -Path $TsvFileName -NoTypeInformation
}
}
}
}
}
function ConvertTo-CleartextPassword
{
<#
.SYNOPSIS
This function can be used to decrypt passwords that were stored encrypted by the function Invoke-PasswordRoll.
Function: ConvertTo-CleartextPassword
Author: Microsoft
Version: 1.0
.DESCRIPTION
This function can be used to decrypt passwords that were stored encrypted by the function Invoke-PasswordRoll.
.PARAMETER EncryptedPassword
The encrypted password that was stored in a TSV file.
.PARAMETER EncryptionKey
The password used to do the encryption.
.EXAMPLE
. .\Invoke-PasswordRoll.ps1 #Loads the functions in this script file
ConvertTo-CleartextPassword -EncryptionKey "Password1" -EncryptedPassword
76492d1116743f0423413b16050a5345MgB8AGcAZgBaAHUAaQBwADAAQgB2AGgAcABNADMASwBaAFoAQQBzADEAeABjAEEAPQA9AHwAZgBiAGYA
MAA1ADYANgA2ADEANwBkADQAZgAwADMANABjAGUAZQAxAGIAMABiADkANgBiADkAMAA4ADcANwBhADMAYQA3AGYAOABkADcAMQA5ADQAMwBmAGYA
NQBhADEAYQBjADcANABkADIANgBhADUANwBlADgAMAAyADQANgA1ADIAOQA0AGMAZQA0ADEAMwAzADcANQAyADUANAAzADYAMAA1AGEANgAzADEA
MQA5ADAAYwBmADQAZAA2AGQA"
Decrypts the encrypted password which was stored in the TSV file.
#>
Param(
[Parameter(Mandatory=$true)]
[String]
$EncryptedPassword,
[Parameter(Mandatory=$true)]
[String]
$EncryptionKey
)
$Sha256 = new-object System.Security.Cryptography.SHA256CryptoServiceProvider
$SecureStringKey = $Sha256.ComputeHash([System.Text.UnicodeEncoding]::Unicode.GetBytes($EncryptionKey))
[SecureString]$SecureStringPassword = ConvertTo-SecureString -String $EncryptedPassword -Key
$SecureStringKey
Write-Output ([System.Runtime.InteropServices.Marshal]::PtrToStringAuto
([System.Runtime.InteropServices.Marshal]::SecureStringToCoTaskMemUnicode($SecureStringPassword)))
}
當我使用以下命令運行腳本時:
Invoke-PasswordRoll -ComputerName (Get-Content computerlist.txt) -LocalAccounts @("administrator","CustomLocalAdmin") -TsvFileName "LocalAdminCredentials.tsv" -EncryptionKey "Password1"
它會變更目標電腦上的密碼,但不會將其變更為「Password1」。這個腳本到底在做什麼?
答案1
就像Tomasz和Zoredache所說:微軟提供的腳本只能用於將遠端機器上的本機帳戶密碼設定為隨機密碼。可以使用管理員選擇的密碼(參數 )對儲存在 CSV 檔案中的帳戶密碼進行加密,-EncryptionKey
以確保明文帳戶密碼不會寫入磁碟。
加密的密碼(儲存在 TSV 檔案中)可以使用同一檔案中的另一個函數進行解密:ConvertTo-CleartextPassword。
在我們的環境中,我們還希望定期更改密碼,因此我們創建了兩個腳本:一個用於使用加密密碼(我們自己選擇的)創建一個新文件,並將該文件分發到我們所有的工作站。秒腳本(也分發到工作站)每 X 小時執行一次,並根據檔案的內容重設密碼。當然,用於加密和解密的密碼仍然以明文形式存儲,但密碼本身不是。這樣,您確實需要知道您正在做什麼來檢索本機管理員密碼,因為加密檔案和腳本保存在不同的位置,因此非常安全。
建立密碼文件
我們使用以下腳本(我刪除了錯誤日誌記錄和 try/catches)來建立密碼檔案:
$LocalAdminPW = "0urAdminP@ssword" #the password that is used to set as the local admin password
$EncryptionPW = "0urEncryptionP@ssword" #password to encrypt and decrypt the password
$File = "C:\Temp\Password.tsv" #file to create
#Create encryption key
$Sha256 = new-object System.Security.Cryptography.SHA256CryptoServiceProvider
$SecureStringKey = $Sha256.ComputeHash([System.Text.UnicodeEncoding]::Unicode.GetBytes($EncryptionPW))
#Encrypt the password with the user supplied encryption password
PasswordSecureString = ConvertTo-SecureString -AsPlainText -Force -String $LocalAdminPW
#Create TSV-File with the encrypted password
ConvertFrom-SecureString -Key $SecureStringKey -SecureString $PasswordSecureString | Out-File -Force -FilePath "$File"
#Gathering the new encrypted password for the local administrator account
$LocalAdminPW = Get-Content $FileLocation
使用建立的密碼檔案設定本機管理員密碼
我們使用以下腳本從密碼檔案中解密密碼,並使用它來設定管理員密碼(我刪除了錯誤處理和日誌記錄):
#function to get decrypted password
Function Get-DecryptedPassword {
[CmdletBinding()]
param(
$EncryptedPW,
$LocalAdminPW
)
#Decrypt the password with the user supplied encryption password
$Sha256 = new-object System.Security.Cryptography.SHA256CryptoServiceProvider
$SecureStringKey = $Sha256.ComputeHash([System.Text.UnicodeEncoding]::Unicode.GetBytes($EncryptionPW))
[SecureString]$SecureStringPassword = ConvertTo-SecureString -String $LocalAdminPW -Key $SecureStringKey
return ([System.Runtime.InteropServices.Marshal]::PtrToStringAuto([System.Runtime.InteropServices.Marshal]::SecureStringToCoTaskMemUnicode($SecureStringPassword)))
}
#Change password local admin account
$LocalAdmin = "Administrator"
$objUser = [ADSI]"WinNT://localhost/$($LocalAdmin), user"
$objUser.psbase.Invoke("SetPassword", (Get-DecryptedPassword -EncryptedPW $EncryptionPW -LocalAdminPW $LocalAdminPW))
答案2
將密碼儲存在電子表格中並不是最佳選擇,並且將所有電腦設定為相同的密碼是憑證盜竊的另一個問題(即使您定期更改密碼)。 LAPS 工具可用於安全地隨機化本機管理員密碼。如果您嘗試解決本文中的問題,請考慮使用網域憑證。如果您只是想更改密碼,請使用 LAPS 工具(https://technet.microsoft.com/en-us/library/security/3062591.aspx)
答案3
只需閱讀貼上腳本中的評論:
如果系統上存在本機帳戶“administrator”和/或“CustomLocalAdmin”,則其密碼將變更為隨機產生的長度為 20 的密碼(預設值)。
使用者名稱/密碼/伺服器組合儲存在 LocalAdminCredentials.tsv 中,帳戶密碼使用密碼「Password1」進行 AES 加密。